Code
= c(1, 2, 3, 4)
a a
[1] 1 2 3 4
::: {.cell}
:::
A vector is an ordered collection of basic data types of a given length. The only key thing here is all the elements of a vector must be same data type e.g homogeneous data structures. Vectors are one-dimensional data structures.
create 5 random number from 1 to 10 without replacement
create 5 random number from 1 to 10 with replacement
create 1 random number from 0 to 1 from random uniform distribution
generate 4 random number that follows the normal distribution with mean being 0 and standard deviation being 1
or
or
find number only in xx not in yy
Name Marks
1 Jhon 56
2 Lee 76
3 Suzan 86
4 Abhinav 96
5 Brain 73
6 Emma 87
7 David 47
8 Alice 98
other way: ::: {.cell}
[,1] [,2]
[1,] 56 56
[2,] 76 76
[3,] 86 86
[4,] 96 96
[5,] 73 73
[6,] 87 87
[7,] 47 47
[8,] 98 98
:::
each element in vetor as a element in list
3D array ::: {.cell}
[1] "array"
:::
Dataframes are generic data objects of R which are used to store the tabular data. Dataframes are the foremost popular data objects in R programming because we are comfortable in seeing the data within the tabular form. They are two-dimensional, heterogeneous data structures
# A vector which is a character vector
Name = c("Amiya", "Raj", "Asish")
# A vector which is a character vector
Language = c("R", "Python", "Java")
# A vector which is a numeric vector
Age = c(22, 25, 45)
# To create dataframe use data.frame command
# and then pass each of the vectors
# we have created as arguments
# to the function data.frame()
df = data.frame(Name, Language, Age)
df
Name Language Age
1 Amiya R 22
2 Raj Python 25
3 Asish Java 45
A matrix is a rectangular arrangement of numbers in rows and columns. In a matrix, as we know rows are the ones that run horizontally and columns are the ones that run vertically. Matrices are two-dimensional, homogeneous data structures.
[,1] [,2] [,3]
[1,] 1 2 3
[2,] 4 5 6
[3,] 7 8 9
A list is a generic object consisting of an ordered collection of objects. Lists are heterogeneous data structures. These are also one-dimensional data structures. A list can be a list of vectors, list of matrices, a list of characters and a list of functions and so on.
empId = c(1, 2, 3, 4)
# The second attribute is the employee name
# which is created using this line of code here
# which is the character vector
empName = c("Debi", "Sandeep", "Subham", "Shiba")
# The third attribute is the number of employees
# which is a single numeric variable.
numberOfEmp = 4
# We can combine all these three different
# data types into a list
# containing the details of employees
# which can be done using a list command
empList = list(empId, empName, numberOfEmp)
empList
[[1]]
[1] 1 2 3 4
[[2]]
[1] "Debi" "Sandeep" "Subham" "Shiba"
[[3]]
[1] 4
3D arrays
, , 1
[,1] [,2]
[1,] 1 3
[2,] 2 4
, , 2
[,1] [,2]
[1,] 5 7
[2,] 6 8
https://www.geeksforgeeks.org/data-structures-in-r-programming/
---
title: "Data structure in R"
execute:
warning: false
error: false
format:
html:
toc: true
toc-location: right
code-fold: show
code-tools: true
number-sections: true
code-block-bg: true
code-block-border-left: "#31BAE9"
---
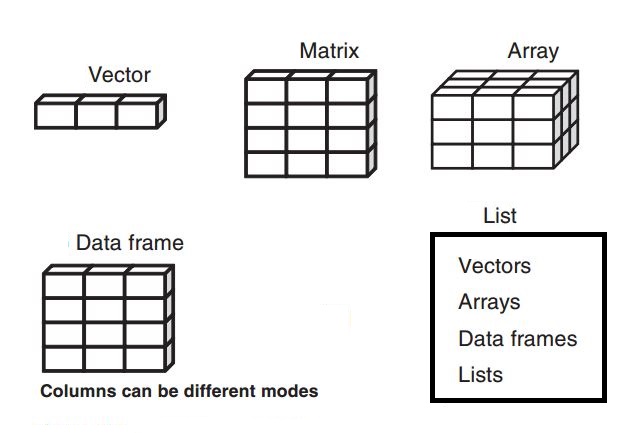{width="600"}
```{r}
library(tidyverse)
```
# vector
A vector is an ordered collection of basic data types of a given length. The only key thing here is all the elements of a vector must be same data type e.g homogeneous data structures. Vectors are one-dimensional data structures.
```{r}
a = c(1, 2, 3, 4)
a
```
```{r}
class(a)
```
```{r}
b = c("Debi", "Sandeep", "Subham", "Shiba")
b
```
```{r}
class(b)
```
## create sequence vector
```{r}
seq(from = 2, to = 14, by = 2)
```
## create repeat vector
```{r}
rep(x = 1.5, times = 4)
```
## create random vector
create 5 random number from 1 to 10 without replacement
```{r}
sample(1:10,5, replace=F)
```
create 5 random number from 1 to 10 with replacement
```{r}
sample(1:10,5, replace=T)
```
create 1 random number from 0 to 1 from random uniform distribution
```{r}
runif(1, min=0, max=1)
```
generate 4 random number that follows the normal distribution with mean being 0 and standard deviation being 1
```{r}
sn1 <- rnorm(4, mean=0, sd=1) # standard nromal
sn1
```
## create unique vector
```{r}
v1=c(1,1,2,2,5,6)
v1
```
```{r}
unique(v1)
```
## append vector
```{r}
x=c(1,2,3)
y=c(4,5,6)
z=c(x,y)
z
```
## remove element in vector
```{r}
x=c(1,2,3,4,5)
x
```
### remove first one
```{r}
x[-1]
```
### remove last one
```{r}
x[-length(x)]
```
### remove from last second
```{r}
x[1:(length(x)-2)]
```
### remove from from another vector
```{r}
remove=c(2,4)
x[-remove]
```
## sort vector
```{r}
a=c(2,4,6,1,4)
```
```{r}
sort(a)
```
```{r}
sort(a,decreasing=TRUE)
```
## vector length
```{r}
length(a)
```
## calculate vector
```{r}
x=c(1,2,3,4,5)
sum(x)
```
## select vector element
```{r}
x=c(1,2,3,6,9,10)
```
### select first
```{r}
x[1]
```
or
```{r}
x %>% nth(1)
```
### select last
```{r}
x %>% last()
```
or
```{r}
x %>% nth(-1)
```
### select second last
```{r}
x %>% nth(-2)
```
### select first to 3th
```{r}
x[1:3]
```
### select last one to last 3th
```{r}
x[-3:-1]
```
## compare two vector
```{r}
xx=c(1,2,3,4)
xx
```
```{r}
yy=c(2,4)
yy
```
find number only in xx not in yy
```{r}
setdiff(xx, yy)
```
## vector Converting between types
### to factor
```{r}
x <- c("a", "g", "b")
y=as.factor(x)
y
```
### to numeric
```{r}
x <- c('123','44', '222')
y=as.numeric(x)
y
```
### to character
```{r}
x <- c(123123,111,5555)
y=as.character(x)
y
```
### to boolen
```{r}
x <- c(1,0,1)
y=as.logical(x)
y
```
## vector to other data format
### vector to dataframe
```{r}
Name <- c("Jhon", "Lee", "Suzan", "Abhinav",
"Brain", "Emma", "David", "Alice")
Marks <- c(56, 76, 86, 96, 73, 87, 47, 98)
data<- data.frame(Name,Marks)
data
```
```{r}
class(data)
```
### verctor to matrix
```{r}
v1 <- c(56, 76, 86, 96, 73, 87, 47, 98)
```
```{r}
data<-matrix(v1,nrow=4)
data
```
```{r}
class(data)
```
other way:
```{r}
data<-matrix(c(v1,v1),ncol = 2)
data
```
### verctor to list
```{r}
data=list(v1)
data
```
```{r}
class(data)
```
each element in vetor as a element in list
```{r}
data=as.list(v1)
data
```
### verctor to array
3D array
```{r}
vec1=c(1:5)
vec2=c(6:10)
arr=array(c(vec1,vec2),dim=c(2,5,3))
# printing the array
class(arr)
```
```{r}
arr
```
# Dataframe
Dataframes are generic data objects of R which are used to store the tabular data. Dataframes are the foremost popular data objects in R programming because we are comfortable in seeing the data within the tabular form. They are two-dimensional, heterogeneous data structures
```{r}
# A vector which is a character vector
Name = c("Amiya", "Raj", "Asish")
# A vector which is a character vector
Language = c("R", "Python", "Java")
# A vector which is a numeric vector
Age = c(22, 25, 45)
# To create dataframe use data.frame command
# and then pass each of the vectors
# we have created as arguments
# to the function data.frame()
df = data.frame(Name, Language, Age)
df
```
```{r}
class(df)
```
## dataframe to other data format
### dataframe to matrix
```{r}
mat <- as.matrix(df)
class(mat)
```
```{r}
mat
```
### dataframe to vector
```{r}
vec=df[['Name']]
class(vec)
```
```{r}
vec
```
### dataframe to list
```{r}
list=as.list(df[['Name']])
class(list)
```
```{r}
list
```
# Matrices
A matrix is a rectangular arrangement of numbers in rows and columns. In a matrix, as we know rows are the ones that run horizontally and columns are the ones that run vertically. Matrices are two-dimensional, homogeneous data structures.
```{r}
A = matrix(
# Taking sequence of elements
c(1, 2, 3, 4, 5, 6, 7, 8, 9),
# No of rows and columns
nrow = 3, ncol = 3,
# By default matrices are
# in column-wise order
# So this parameter decides
# how to arrange the matrix
byrow = TRUE
)
A
```
```{r}
class(A)
```
```{r}
matrix002=A+A
matrix002
```
```{r}
matrix003=A*A
matrix003
```
## matrix to other data format
### matrix to dataframe
```{r}
df <- as.data.frame(matrix003)
class(df)
```
```{r}
df
```
### matrix to vector
```{r}
vec=as.vector(matrix003)
class(vec)
```
```{r}
vec
```
### matrix to list
```{r}
list=as.list(matrix003)
class(list)
```
```{r}
list
```
# Lists
A list is a generic object consisting of an ordered collection of objects. Lists are heterogeneous data structures. These are also one-dimensional data structures. A list can be a list of vectors, list of matrices, a list of characters and a list of functions and so on.
```{r}
empId = c(1, 2, 3, 4)
# The second attribute is the employee name
# which is created using this line of code here
# which is the character vector
empName = c("Debi", "Sandeep", "Subham", "Shiba")
# The third attribute is the number of employees
# which is a single numeric variable.
numberOfEmp = 4
# We can combine all these three different
# data types into a list
# containing the details of employees
# which can be done using a list command
empList = list(empId, empName, numberOfEmp)
empList
```
```{r}
class(empList)
```
# Arrays
3D arrays
```{r}
A = array(
# Taking sequence of elements
c(1, 2, 3, 4, 5, 6, 7, 8),
# Creating two rectangular matrices
# each with two rows and two columns
dim = c(2, 2, 2)
)
A
```
```{r}
class(A)
```
# Reference:
https://www.geeksforgeeks.org/data-structures-in-r-programming/